Python 常用字符串方法
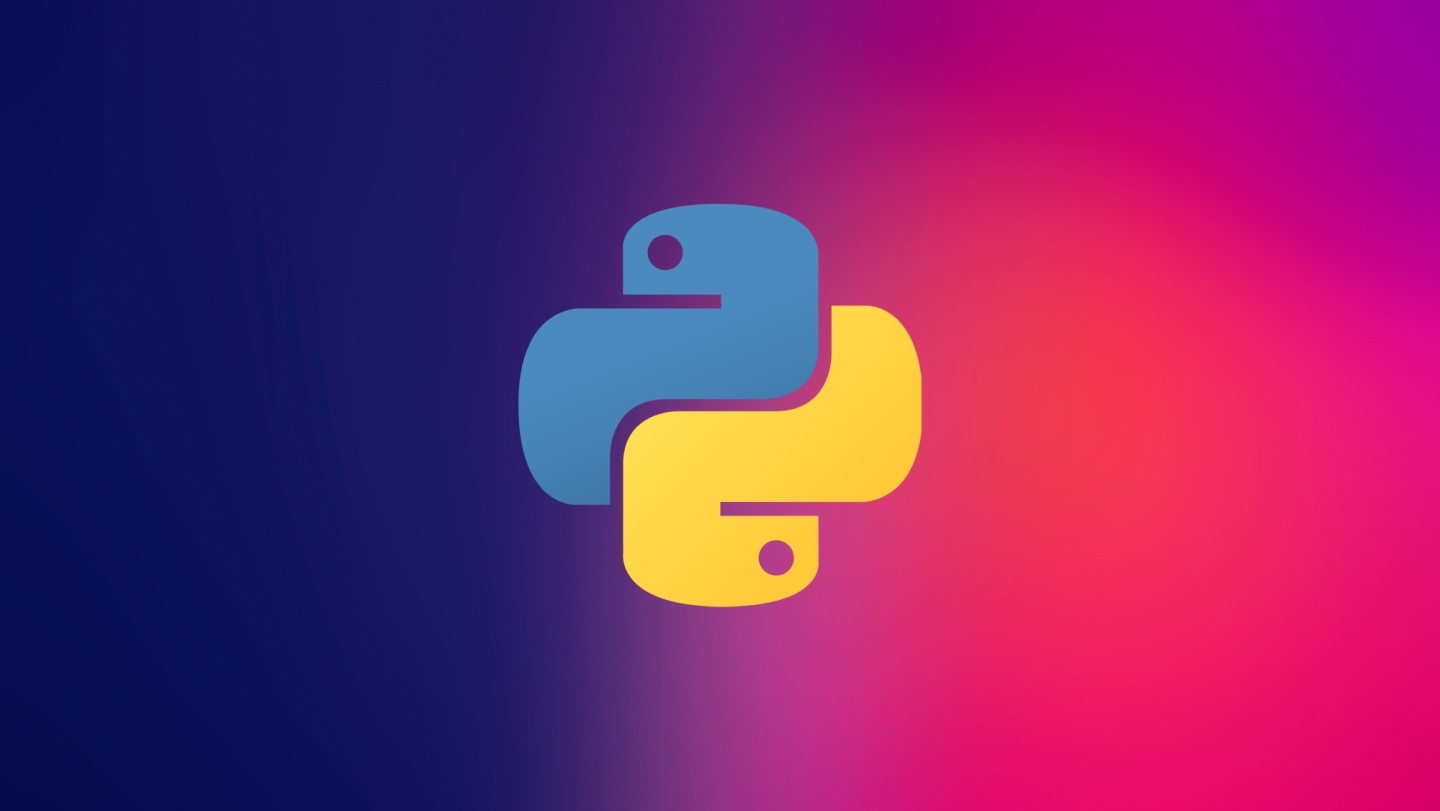
AI-摘要
小嗷犬 GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
Python 常用字符串方法
小嗷犬获取字符串长度函数 len()
len()
函数可以帮助我们获取字符串长度,即字符串中字符的个数:
1 |
|
字符串的 in 和 not in 操作符
in
和not in
操作符可以用于第一个字符串(大小写敏感)是否在第二个字符串中,返回一个 bool 类型的值:
1 |
|
字符串方法 upper()、lower()
upper()
和lower()
字符串方法返回一个新字符串,其中原字符串的所有字母都被相应地转换为大写或小写。字符串中非字母字符保持不变。
1 |
|
字符串方法 isX()
Python中有许多判断字符串的isX()
方法:
isupper()
,如果字符串含有字母,并且所有字母都是大写,返回True;islower()
,如果字符串含有字母,并且所有字母都是小写,返回True;isalpha()
,如果字符串只包含字母和汉字,并且非空,返回True;isalnum()
,如果字符串只包含字母、汉字和数字,并且非空,返回True;isdecimal()
,如果字符串只包含数字字符,并且非空,返回True;isspace()
,如果字符串只包含空格、制表符和换行,并且非空,返回True;istitle()
,如果字符串仅包含以大写字母开头、后面都是小写字母的单词,返回True。
1 |
|
字符串方法 startswith() 和 endswith()
startswith()
和endswith()
,如果它们所调用的字符串以该方法传入的字符串开始或结束,返回True
。否则,返回False
。经常被用于判断网址链接或是文件类型后缀:
1 |
|
字符串方法 join() 和 split()
join()
方法用于将一个字符串列表用特定的字符串连接起来,返回连接后的字符串,基本使用格式:
1 |
|
如:
1 |
|
split()
则完全相反,它会按照指定的字符串来将原本的字符串切割成字符串列表,返回生成的列表,基本使用格式:
1 |
|
如:
1 |
|
字符串方法 rjust()、ljust() 和 center()
rjust()
和ljust()
字符串方法返回调用它们的字符串的填充版本,通过插入空格来对齐文本。这两个方法的第一个参数是一个整数长度,用于对齐字符串,第二个可选参数将指定一个填充字符,取代空格字符。
center()
字符串方法则是让文本居中,而不是左对齐或右对齐。
1 |
|
字符串方法 strip()、rstrip() 和 lstrip()
有时候你希望删除字符串左边、右边或两边的空白字符(空格、制表符和换行符),可以使用这些方法:
strip()
方法将返回一个新的字符串,它的开头或末尾都没有空白字符。lstrip()
方法将相应删除左边的空白字符。rstrip()
方法将相应删除右边的空白字符。
1 |
|
这三个方法还可以传入一个字符串参数,指定要删除的字符:
1 |
|
字符串方法 replace()
replace()
方法用于将字符串中的所有指定字符串替换为另一个指定的字符串,返回替换后的字符串:
1 |
|
评论
隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果