⎨⎧x12−x2+x32≥0x1+x22+x33≤20−x1−x22+2=0x2+2x32=3x1,x2,x3≥0解
转换为标准形式:
minf(x)=x12+x22+x32+8
s.t. ⎩⎨⎧−x12+x2−x32≤0x1+x22+x33−20≤0x1+x22−2=0x2+2x32−3=0x1,x2,x3≥0
定义目标函数:
1 2 3
| function f = objfun(x) f = x(1)^2 + x(2)^2 + x(3)^2 + 8; end
|
定义非线性约束函数:
1 2 3 4
| function [c,ceq] = nonlcon(x) c = [-x(1)^2 + x(2) - x(3)^2; x(1) + x(2)^2 + x(3)^3 - 20]; ceq = [x(1) + x(2)^2 - 2; x(2) + 2*x(3)^2 - 3]; end
|
代码求解:
1
| [x,fval] = ga(@objfun,3,[],[],[],[],[0,0,0],[],@nonlcon)
|
输出结果:
1 2 3 4 5
| x = 0.5516 1.2035 0.9477
fval = 10.6508
|
例2
求解以下整数规划问题:
maxZ=4x1+3y1+5y2
s.t. ⎩⎨⎧y1,y2 are integers2x1+y1+3y2≤36x1+y1≥8x1+y2≥10x1+y1−y2=4x1,y1,y2≥0
解
转换为标准形式:
min−Z=−4x1−3y1−5y2
s.t. ⎩⎨⎧y1,y2 are integers2x1+y1+3y2≤36−x1−y1≤−8−x1−y2≤−10x1+y1−y2=4x1,y1,y2≥0
代码求解:
1 2 3 4 5 6 7 8 9 10
| fun = @(x) -4*x(1) - 3*x(2) - 5*x(3); A = [2, 1, 3; -1, -1, 0; -1, 0, -1]; b = [36; -8; -10]; Aeq = [1, 1, -1]; beq = 4; lb = [0, 0, 0]; ub = []; intcon = [2, 3]; [x,fval] = ga(fun,3,A,b,Aeq,beq,lb,ub,[],intcon); fval = -fval;
|
输出结果:
1 2 3 4 5
| x = 4.0000 7.0000 7.0000
fval = 72.0000
|
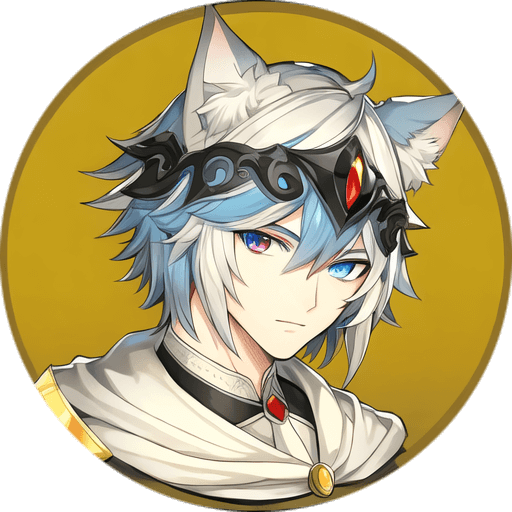
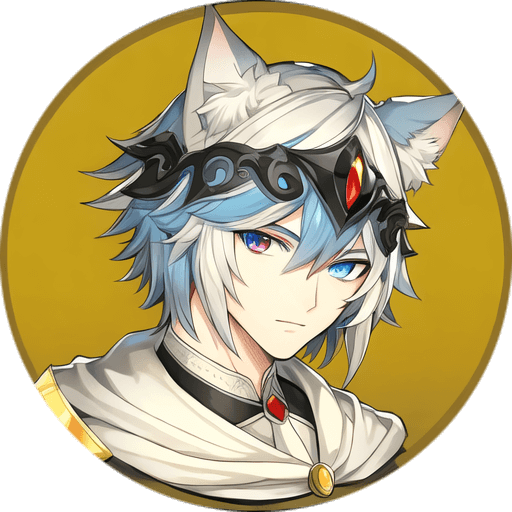
小嗷犬
分享技术,记录生活
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 小嗷犬!